1D Linear Advection#
This problem solves the 1D linear advection
for a scalar field \(\phi\) and advection velocity \(a\).
Initial condition: \(\phi(x, 0) = \sin(\pi x)\)
Domain is \([-1,1]\) with periodic BC
Integration is typically performed over \(t \in (0, 2k)\) where \(k \in \mathbb{Z}\)
Default setting: \(a=1\)
Mesh#
python3 pressio-demoapps/meshing_scripts/create_full_mesh_for.py \
--problem linadv1d_s<stencilSize> -n <N> --outDir <destination-path>
where:
N
is the number of cells you want<stencilSize> = 3 or 5 or 7
: defines the neighboring connectivity of each cell<destination-path>
: full path to where you want the mesh files to be generated. The script creates the directory if it does not exist.
Important
When you set the <stencilSize>
, keep in mind the following constraints (more on this below):
InviscidFluxReconstruction::FirstOder
requires<stencilSize> >= 3
InviscidFluxReconstruction::Weno3
requires<stencilSize> >= 5
InviscidFluxReconstruction::Weno5
requires<stencilSize> >= 7
C++ synopsis#
#include "pressiodemoapps/advection1d.hpp"
int main(){
namespace pda = pressiodemoapps;
const auto meshObj = pda::load_cellcentered_uniform_mesh_eigen("path-to-mesh");
// 1. using default velocity
const auto probId = pda::Advection1d::PeriodicLinear;
const auto scheme = pda::InviscidFluxReconstruction::FirstOder; //or Weno3, Weno5
auto problem = pda::create_problem_eigen(meshObj, probId, scheme);
// 2. specify a custom velocity
const auto scheme = pda::InviscidFluxReconstruction::FirstOder; //or Weno3, Weno5
auto problem = pda::create_linear_advection_1d_problem_eigen(meshObj, scheme, /*vel*);
}
Python synopsis#
import pressiodemoapps as pda
meshObj = pda.load_cellcentered_uniform_mesh("path-to-mesh")
# 1. using default velocity
probId = pda.Advection1d.PeriodicLinear
scheme = pda.InviscidFluxReconstruction.FirstOrder # or Weno3, Weno5
problem = pda.create_problem(meshObj, probId, scheme)
# 2. specify a custom velocity
scheme = pda.InviscidFluxReconstruction.FirstOrder # or Weno3, Weno5
problem = pda.create_linear_advection_1d_problem(meshObj, scheme, #your_vel)
Sample Solution#
Representative plot showing initial condition and solution at \(t=1\) and \(t=2\), obtained using \(dt = 10^{-3}\), Weno5, Runge-Kutta4 integration with a mesh of \(N=250\) cells.
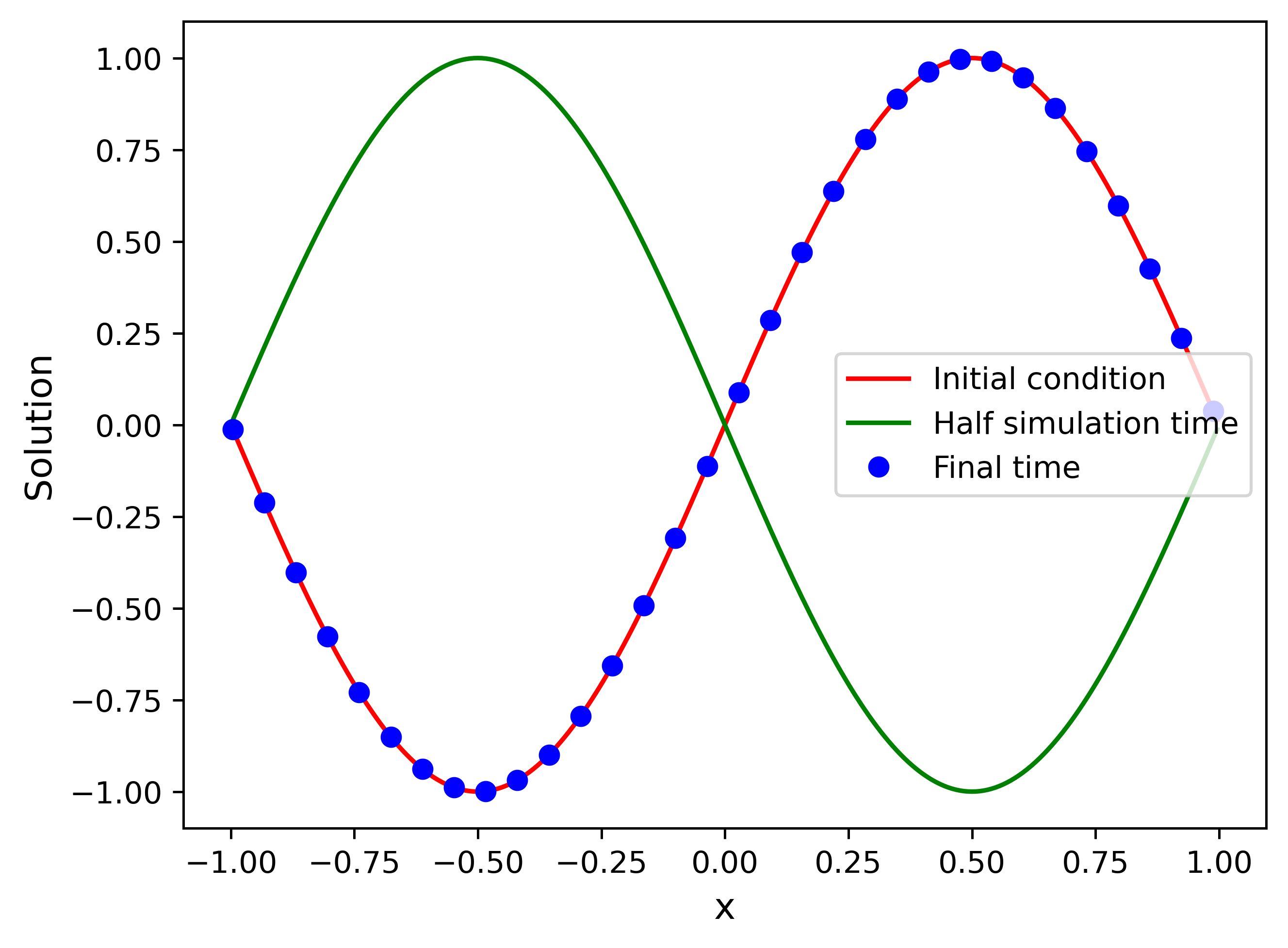